input 값에 대한 validation을 하고 싶을 때,
1. view 쪽에서 API를 호출한다.
2. API는 실패에 대한 메시지를 model에 담아서 보내준다.
3. view 에서는 model에 있는 실패 메세지가 있는지 thymeleaf를 사용해서 처리한다.
요러한 플로우를 통해서 이루어진다고 생각해요. (더 멋지게 구현할수도 있겠지만요...)
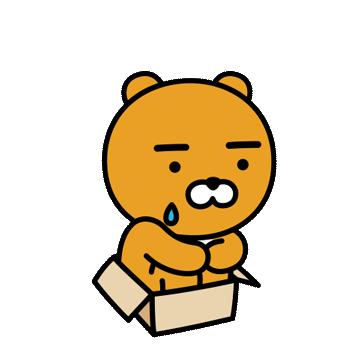
지금은 input 값이 하나라서 다행이지만, 회원가입 같은 여러개의 필드값을 받는다면 어떨까요?
해당 API에서 그 필드값들을 하나씩 분석해서 model에 메세지를 넣어주는건 쉽지 않을거에요.
조금의 설정만 해두면, 위와 같은 문제를 쉽게 해결해줄 수 있는 '@Valid' 가 있습니다!!
@Valid - 클라이언트 측에서 넘어온 데이터를 객체에 바인딩할 때 유효성 검사를 하는 어노테이션
Gradle일 경우 -> build.gradle에 의존성 추가
dependencies{
...
implementation 'org.springframework.boot:spring-boot-starter-validation'
}
Maven일 경우 -> pom.xml에 의존성 추가
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
<version>2.7.4</version>
</dependency>
그 외는 URL 참고
https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-validation/2.7.4
유효성 검사를 위한 어노테이션 적용
어노테이션 종류
Anotation | 제약조건 |
@NotNull | Null 불가 |
@Null | Null만 입력 가능 |
@NotEmpty | Null, 빈 문자열 불가 |
@NotBlank | Null, 빈 문자열, 스페이스만 있는 문자열 불가 |
@Size(min= , max= ) | 문자열, 배열등의 크기가 만족하는가? |
@Pattern(regex= ) | 정규식을 만족하는가? |
@Max(숫자) | 지정 값 이하인가? |
@Min(숫자) | 지정 값 이상인가 |
@Future | 현재 보다 미래인가? |
@Past | 현재 보다 과거인가? |
@Positive | 양수만 가능 |
@PositiveOrZero | 양수와 0만 가능 |
@Negative | 음수만 가능 |
@NegativeOrZero | 음수와 0만 가능 |
이메일 형식만 가능 | |
@Digits(integer= , fraction = ) | 대상 수가 지정된 정수와 소수 자리 수 보다 작은가? |
@DecimalMax(value= ) | 지정된 값(실수) 이하인가? |
@DecimalMin(value= ) | 지정된 값(실수) 이상인가? |
@AssertFalse | false 인가? |
@AssertTrue | true 인가? |
Controller에서 유효성 검사
@GetMapping("/signUpPinNew")
public String signUpPinNew(Model model) {
model.addAttribute("req", new SignUpPinNewDTOReq());
return "member/signUpPinNew";
}
@PostMapping("/signUpPinNew")
public String signUpPinNew(@ModelAttribute("req") @Valid SignUpPinNewDTOReq req, BindingResult result) {
if (result.hasErrors()) {
return "member/signUpPinNew";
}
System.out.println("req.getPinNumber() : " + req.getPinNumber());
return "member/login";
}
- 처음 @Valid를 적용하기 위해 다른 설명글들을 많이 읽어 보았지만, 에러가 많이 났었습니다.
- @Valid의 버전이 문제였던건지 정확힌 문제는 알 수 없었지만 -> 해당 코드는 문제를 해결한 코드 입니다.
- GetMapping에서는 model에 req의 빈객체를 생성해서 줘야 다음 나오는 view코드에서 에러가 안납니다.
- PostMapping에서는 @ModelAttribute -> (" ") 요렇게 작성해주셔야 에러가 안납니다.
View (thymeleaf 코드)
<form action="/member/signUpPinNew" method="post" th:object="${req}">
<input type="number" placeholder="핀번호 (4자리)" th:field="*{pinNumber}" th:class="${#fields.hasErrors('pinNumber')}? 'pinNumber fieldError' : 'pinNumber'"
max="9999" maxlength="4" oninput="numberMaxLength(this);"/>
<div th:if="${#fields.hasErrors('pinNumber')}" th:errors="*{pinNumber}" class="fieldMsgError" style="margin:5px 0 0 10px"></div>
<button class="submitButton submitButtonLabel">핀번호 설정</button>
</form>
- th:object를 사용했기 때문에, 위의 컨트롤러의 GetMapping에서 req의 빈객체를 넣어준것 입니다.
결과 화면
- 참고한 글